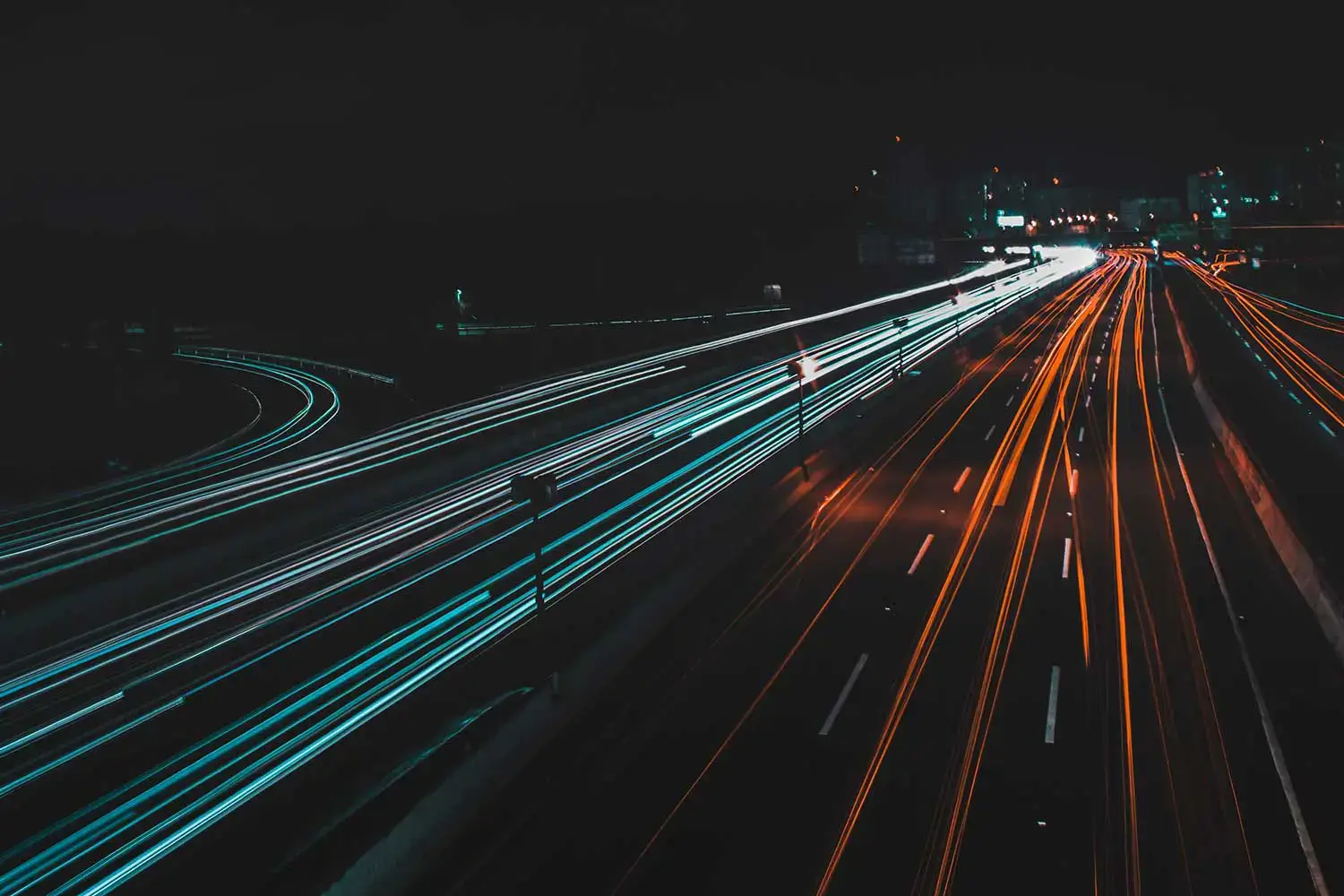
Part of the rendering series
The definition of render blocking means to block the page from continuing to render. When discussing render blocking JavaScript, this can include iframe video embeds, share and social icons/tools, JavaScript libraries, etc.
Google recommends removing or deferring any JavaScript that interferes with loading the content that a user initially sees on your screen when visiting your webpage. That screen could be on a phone, a tablet, a desktop browser or whatever else the end-user may be using to visit your webpage.
As mentioned previously when discussing CSS: any referenced resources will delay your page from loading and rendering for your end-user.
Inline Small JavaScript
Anytime you can include a file’s contents within your HTML you are improving load time because you are saving network calls to external resources. When you inline JavaScript, you are simply including the script file contents within the HTML inside of some script tags. A normal external JavaScript script tag:
<script src="js/scripts.js"></script>
This will make a call to the server to load in the scripts.js file. In doing so, it will halt the loading of the page until the file is loaded. Including the script within the HTML could look like:
<script>
Your JavaScript methods here.
</script>
This will prevent the additional call to the server for resources, but will not necessarily provide the load time improvements that you were expecting. Further improvement can be seen when deferring JavaScript load or asynchronously loading your JavaScript – such as JavaScript libraries.
Ansyc vs. Defer Loading JavaScript
Async
In this context, asynchronous means a process whose execution can proceed independently in the "background". When loading JavaScript asynchronously, the JavaScript file is downloaded while the HTML is parsed. Once the JavaScript download has completed, the HTML parsing will pause in order to execute the JavaScript before the HTML parsing continues.
This is implemented by adding "async" to the script tag. When should you use "async"? If your script is modular and does not rely on any other scripts, you can use async.
<script src="//code.jquery.com/jquery-2.2.4.min.js"></script>
Normal JavaScript Load

<script src="//code.jquery.com/jquery-2.2.4.min.js" async></script>
Asynchronous JavaScript Load

Defer
Most JavaScript is not needed until after page load. Why then do we load the JavaScript before the page has loaded? If your page does not require JavaScript until after it loads, then you can improve your initial page load time by deferring your JavaScript.
We can load our JavaScript after the DOM has completed by relying on the page load event. When the DOM tells us that the page has completed loading, we call a method that appends a script tag to the DOM along with the “src” attribute, essentially only loading the script after the document has completely loaded.
<script src="//code.jquery.com/jquery-2.2.4.min.js"></script>
Normal JavaScript Load

<script src="//code.jquery.com/jquery-2.2.4.min.js" defer></script>
Deferred JavaScript Load

Combine & Minify JavaScript
JavaScript files, like CSS, can be combined into a single file, or a couple of smaller files if necessary. Combining JavaScript files should have little to no effect on how the methods perform, but eliminating references to external files can save you on load time.
The main consideration when putting all of your scripts into a single file is the order of precedence. If your site requires jQuery, you can add it to this single JS file. However, if other methods within your file rely upon jQuery, the library should be included in your file first. Then the scripts that depend on the methods contained within jQuery and so on.
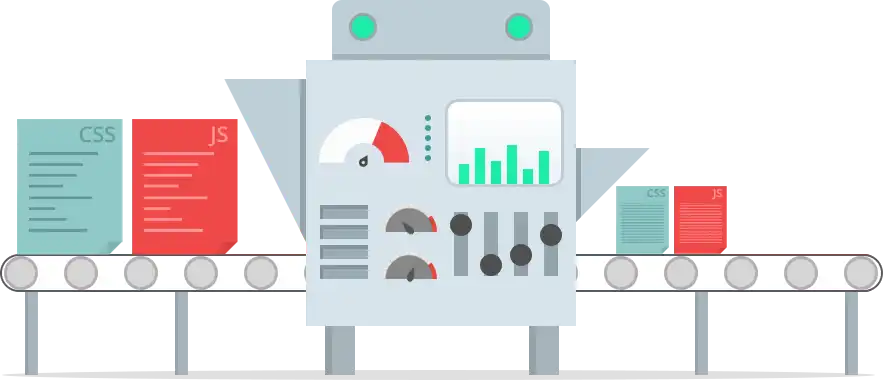